Component communication lesson¶
In this lesson we describe communication of components in more detail.
Application code at start¶
At first check the code basis of the application, this should look like the code resulted in the previous lesson’s The code at the end session.
Adding an inputField¶
Tho be able to discover the communication possibilities of the UI elements we extend the existing DetailPage. At first we add an inputField element to capture new value for the existing DetailPageLabel label.
1page DetailPage {
2 layout: vertical;
3 template: general;
4
5 inputField DetailInputField {
6 template: text;
7 inputType: text;
8 }
9
10 label DetailPageLabel {
11 template: display1Primary;
12 alignment: left;
13 }
14}
The DetailInputField inputField element has attributes with name inputType and template defining the type of the component (check the details in the API documentation).
Binding function to onValueChange event¶
Every time when the content of an inputField is changed an onValueChange event is fired. This event contains the new value of the inputField, so a function has to be bound to this event to be able to capture the new value. To bind a function we can use the createValueChangeSourceEvent() built-in function at the appropriate display() function call as described in the previous lesson, where the extended parameter list of the display() built-in function is used:
1function onNavigationButtonClicked(originId) {
2 var actionEvents = [
3 createValueChangeSourceEvent("DetailInputField", "DetailInputFieldValueChange")
4 ];
5 display(DetailPage, {}, originId, actionEvents);
6}
As final step we can define the logic of function:
1function onDetailInputFieldValueChange(originId) {
2 var value = $IN.data.message;
3}
As you can see the new value of the inputField can be read from the context using $IN.data.message reference.
Changing the value of a label element¶
The behavior of the elements on the UI can be changed using Target Events (check Builder functions section of the API documentation for details).
To change the value of a label element the createValueChangeTargetEvent() function can be used, where the first parameter defines the id of the element, the second defines the new value:
1function onDetailInputFieldValueChange(originId) {
2 fireEvent(createValueChangeTargetEvent("DetailPageLabel", $IN.data.message), originId);
3}
From now on when the user changes the content of the inputField, then the new value is immediately set as value of the label element.
Final form of the code¶
After performing the changes descibed above, the final form of the mobile.scolvo file should be as defined below:
1menu defaultItem HelloWorld {
2 item HelloWorld
3}
4
5function onHelloWorld(originId) {
6 var actionEvents = [
7 buildSourceClickedEvent("NavigationButton", "NavigationButtonClicked")
8 ];
9 display(HelloWorldPage, {}, originId, actionEvents);
10}
11
12page HelloWorldPage {
13 layout: vertical;
14 template: general;
15 settingsVisible: true;
16 scolvoMenuVisible: true;
17
18 label HelloWorldLabel {
19 template: display1Primary;
20 alignment: left;
21 }
22
23 spacer {
24 span: 20;
25 }
26
27 button NavigationButton {
28 template: primary;
29 }
30}
31
32function onHelloWorldPageLoaded(originId) {}
33
34function onNavigationButtonClicked(originId) {
35 var actionEvents = [
36 createValueChangeSourceEvent("DetailInputField", "DetailInputFieldValueChange")
37 ];
38 display(DetailPage, {}, originId, actionEvents);
39}
40
41page DetailPage {
42 layout: vertical;
43 template: general;
44
45 inputField DetailInputField {
46 template: text;
47 inputType: text;
48 }
49
50 label DetailPageLabel {
51 template: display1Primary;
52 alignment: left;
53 }
54}
55
56function onDetailPageLoaded(originId) {}
57
58function onDetailInputFieldValueChange(originId) {
59 fireEvent(createValueChangeTargetEvent("DetailPageLabel", $IN.data.message), originId);
60}
As we have defined new UI elements, the dictionary file of the application has to be extended with the new keys:
1menu_HelloWorld_label=HelloWorld
2page_HelloWorldPage_HelloWorldLabel_defaultText=Hello world!
3page_HelloWorldPage_NavigationButton_label=Display details
4
5page_DetailPage_headerText=Details
6page_DetailPage_DetailInputField_label=Deatils Input
7page_DetailPage_DetailPageLabel_defaultText=Details label
Component communication screen shots¶
The UI resulted of the above code on different client types should look as below:
Component communication - HelloWold page¶
Using browser with light theme
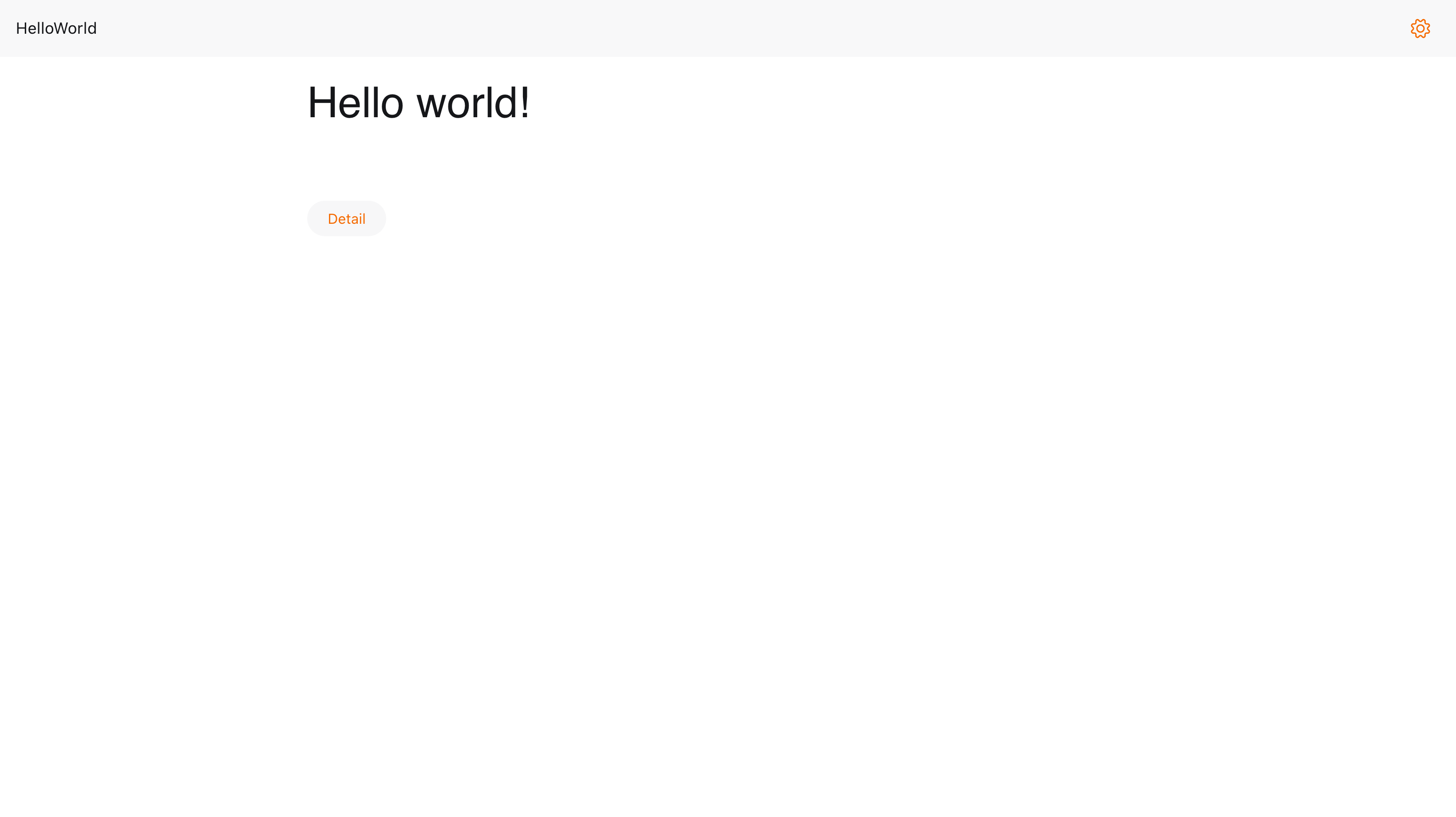
The result of HelloWorld page in browser with light theme
Using browser with dark theme
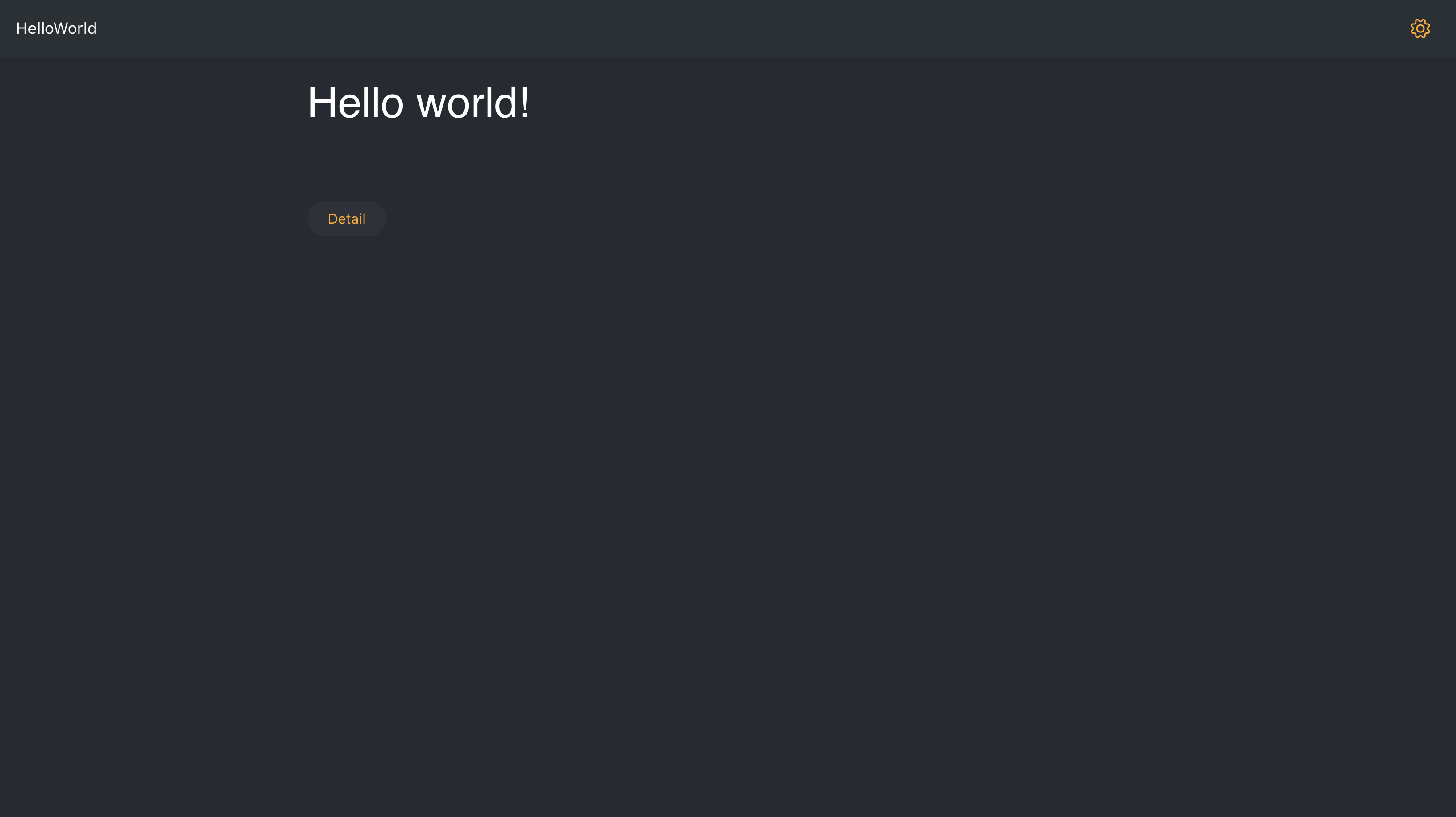
The result of HelloWorld page in browser with dark theme
Using mobile browser with light theme
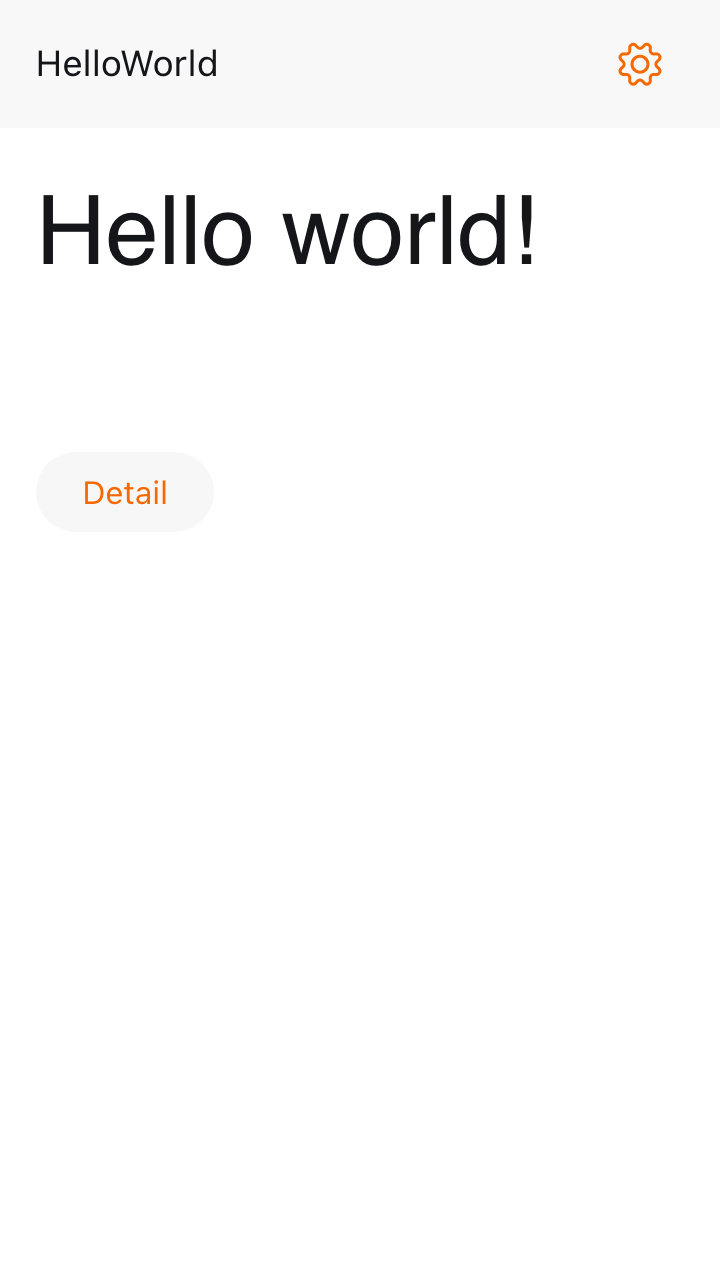
The result of HelloWorld page in mobile browser with light theme
Using mobile browser with dark theme
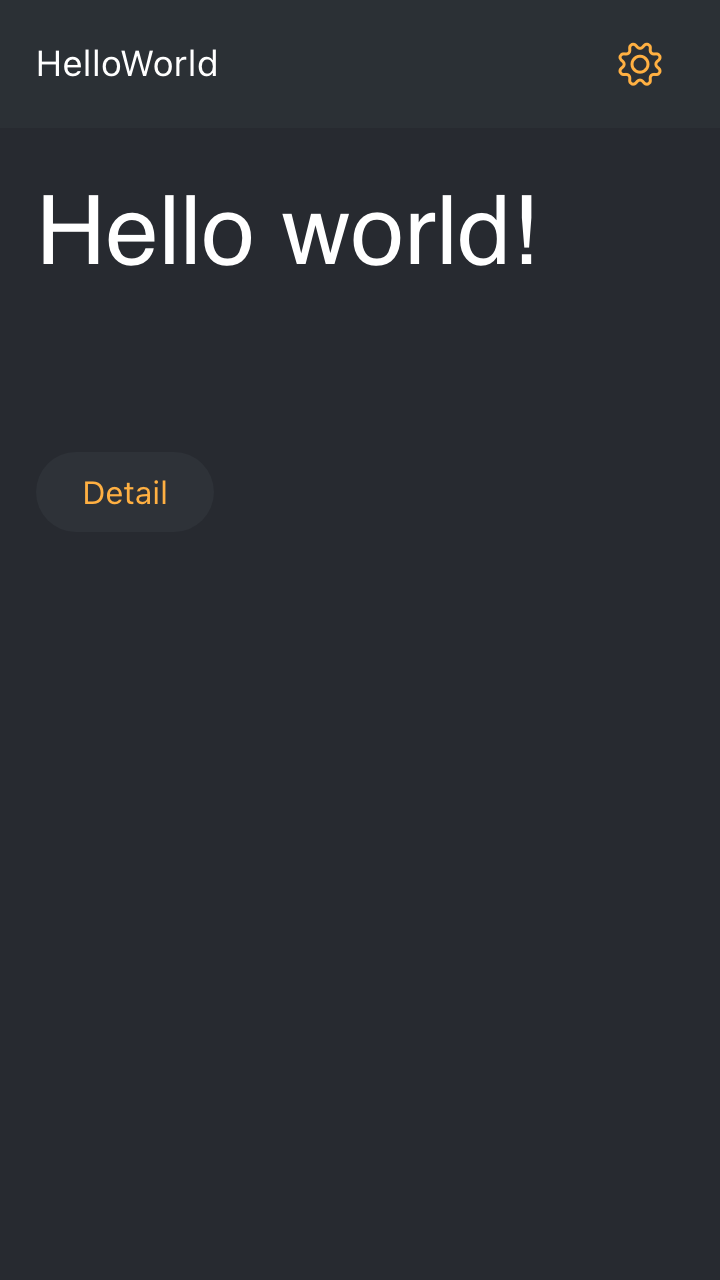
The result of HelloWorld page in mobile browser with dark theme
Using mobile with light theme
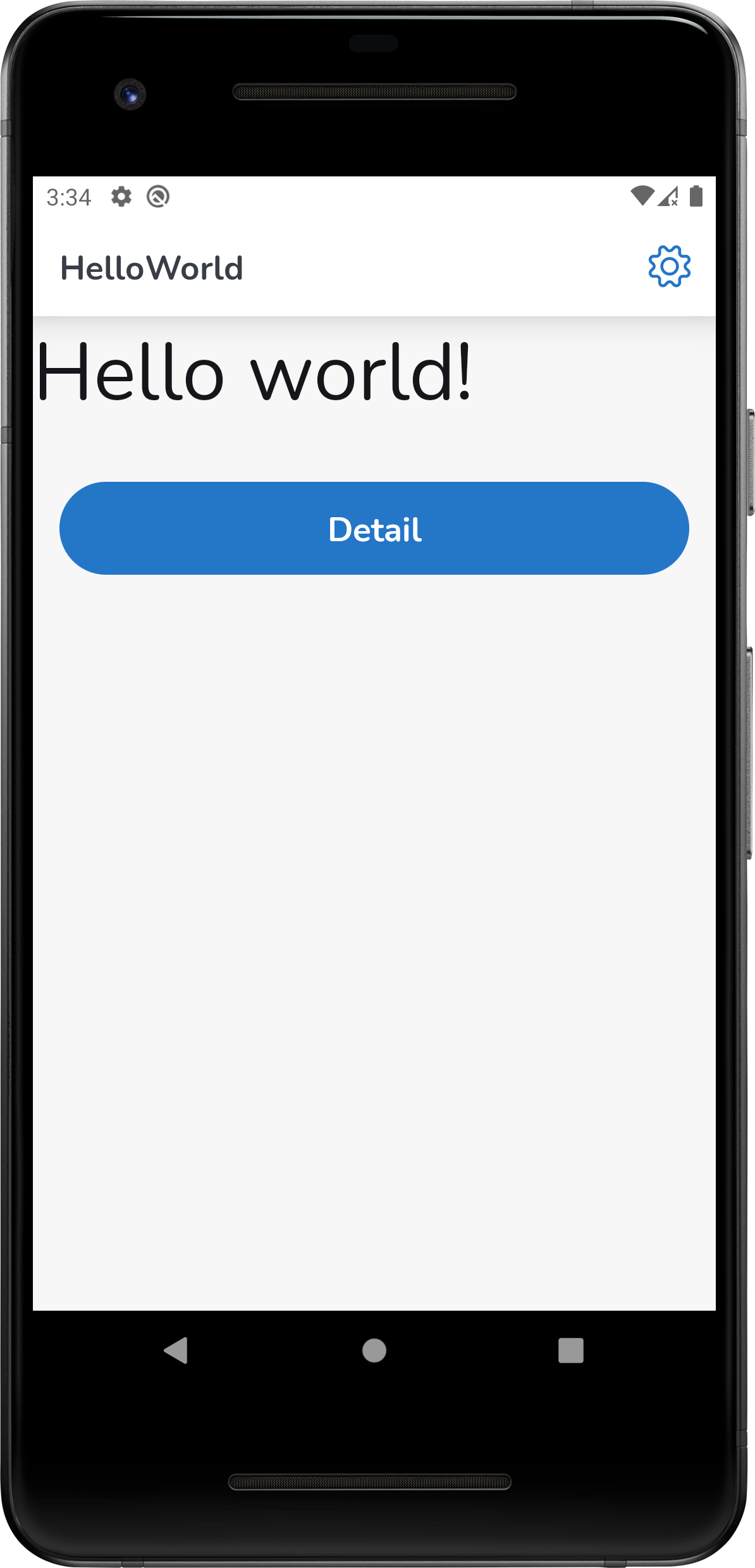
The result of HelloWorld page in mobile with light theme
Using mobile with dark theme
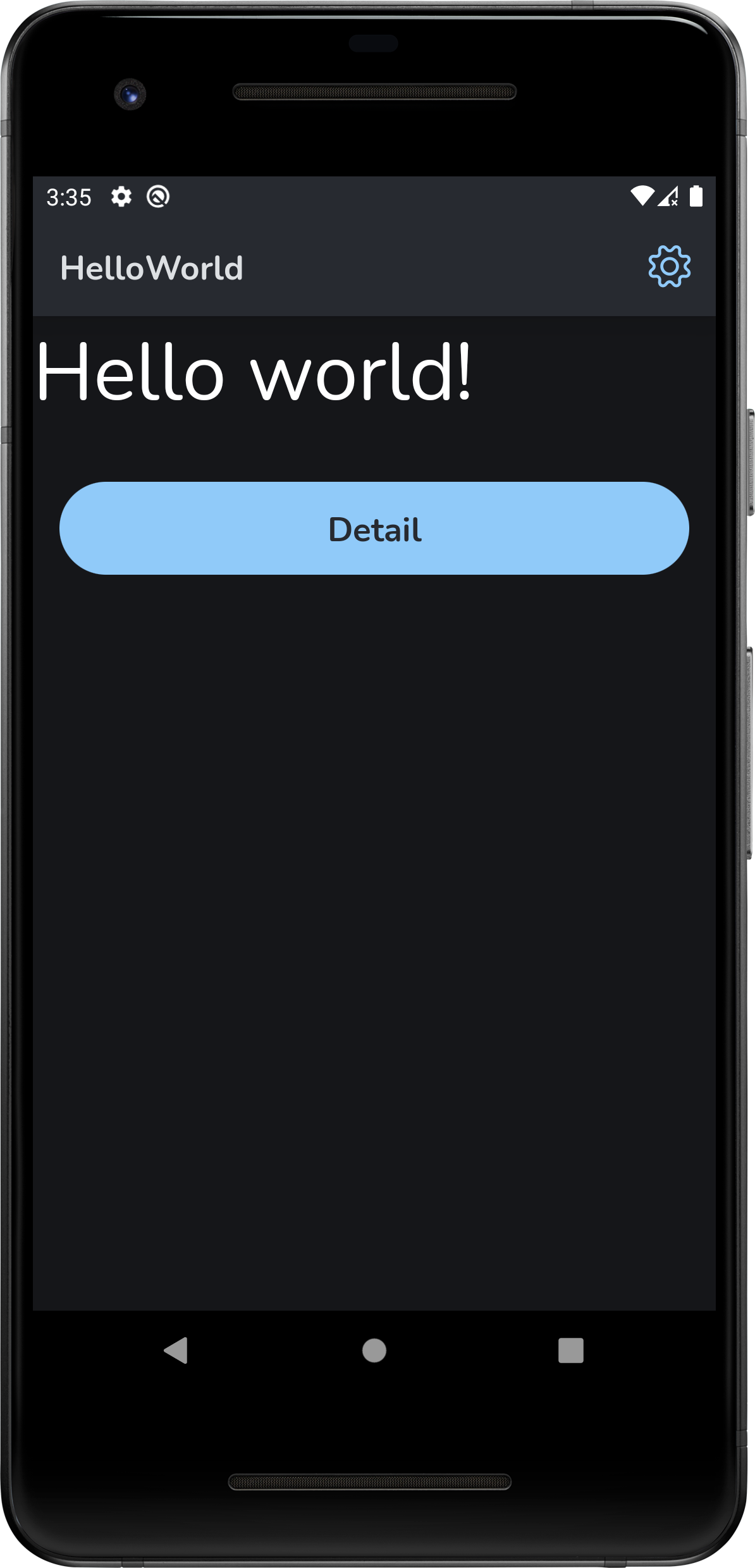
The result of HelloWorld page in mobile with dark theme
Component communication - Detail page¶
Using browser with light theme
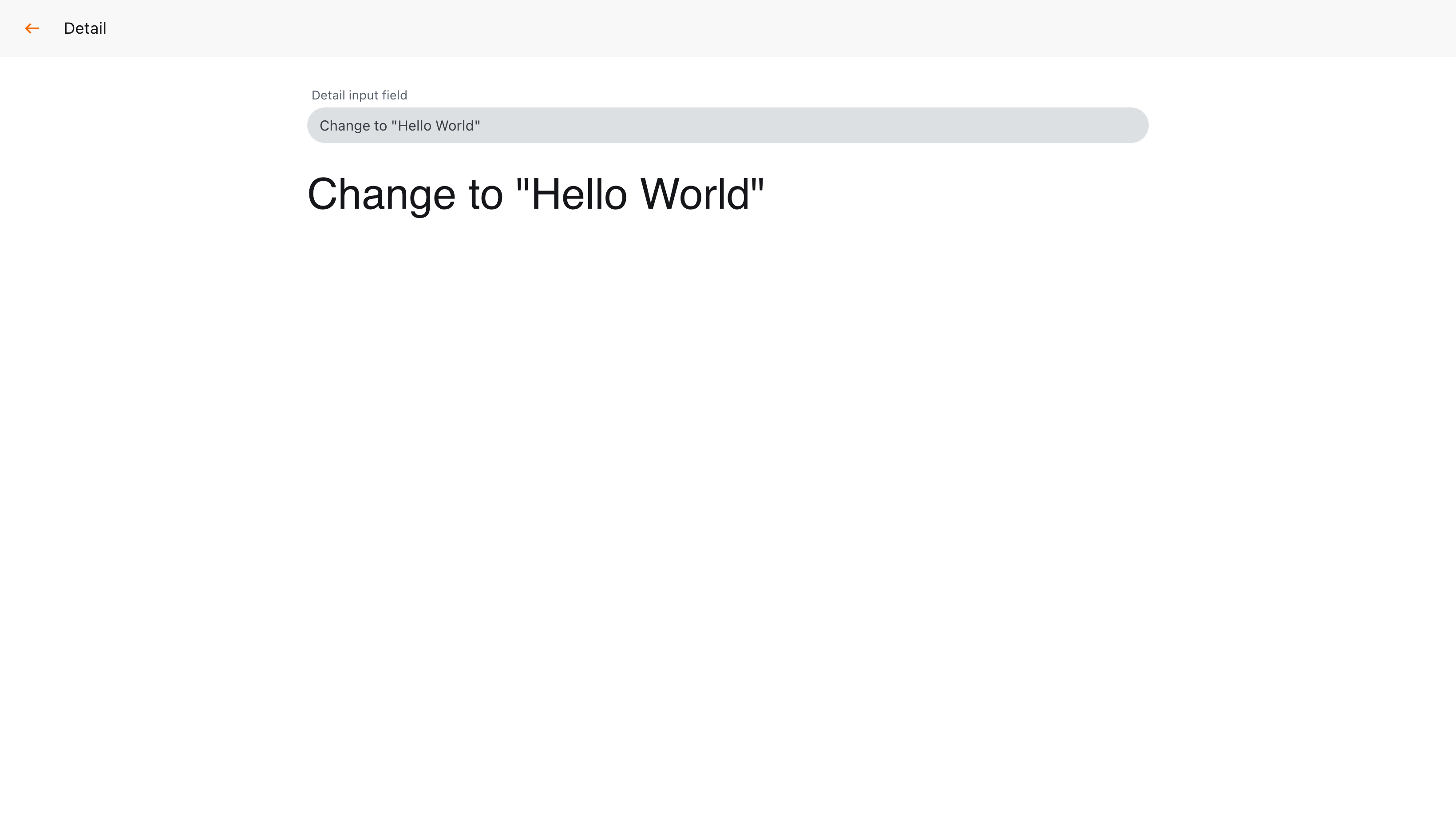
The result of Detail page in browser with light theme
Using browser with dark theme
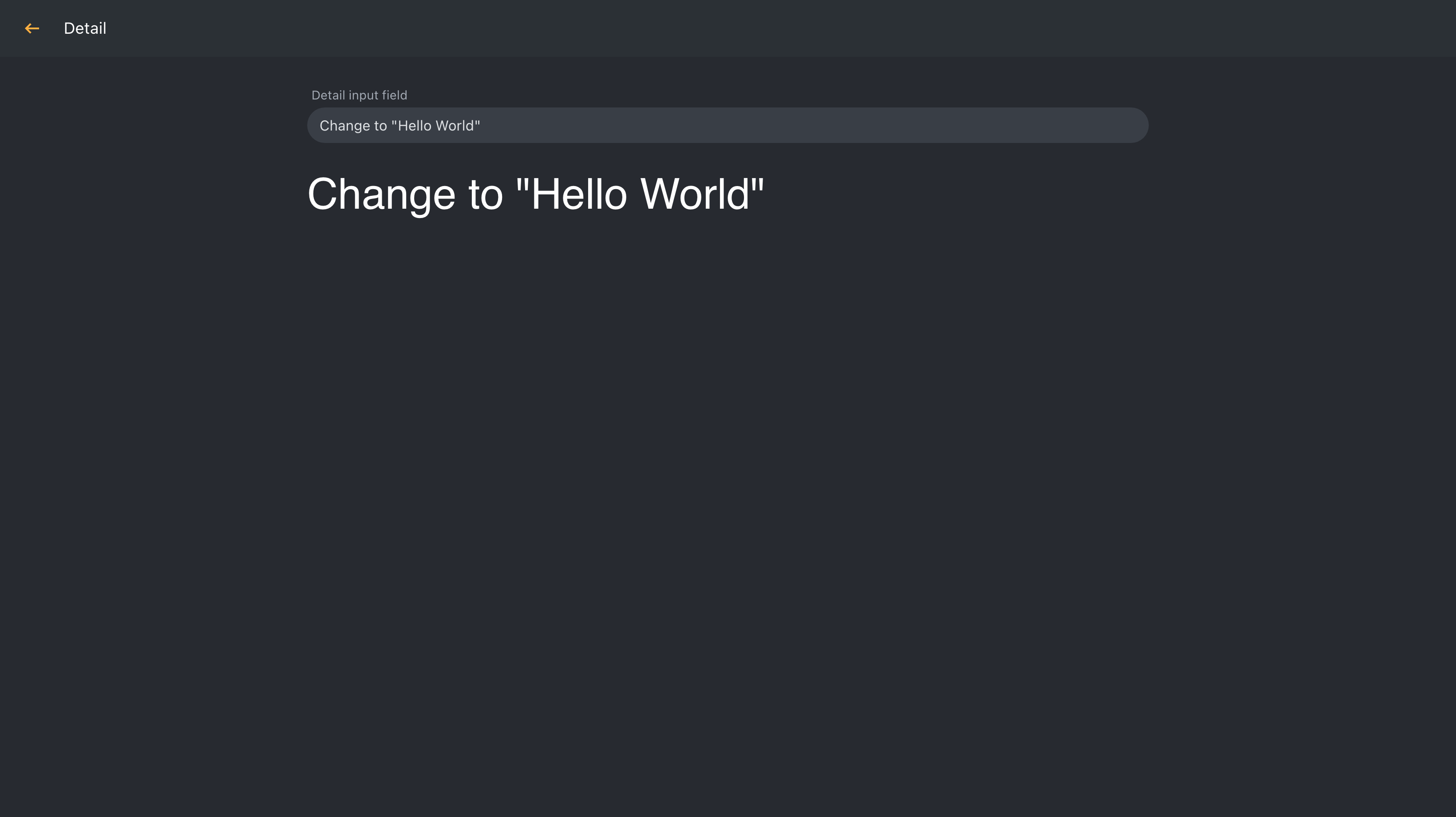
The result of Detail page in browser with dark theme
Using mobile browser with light theme
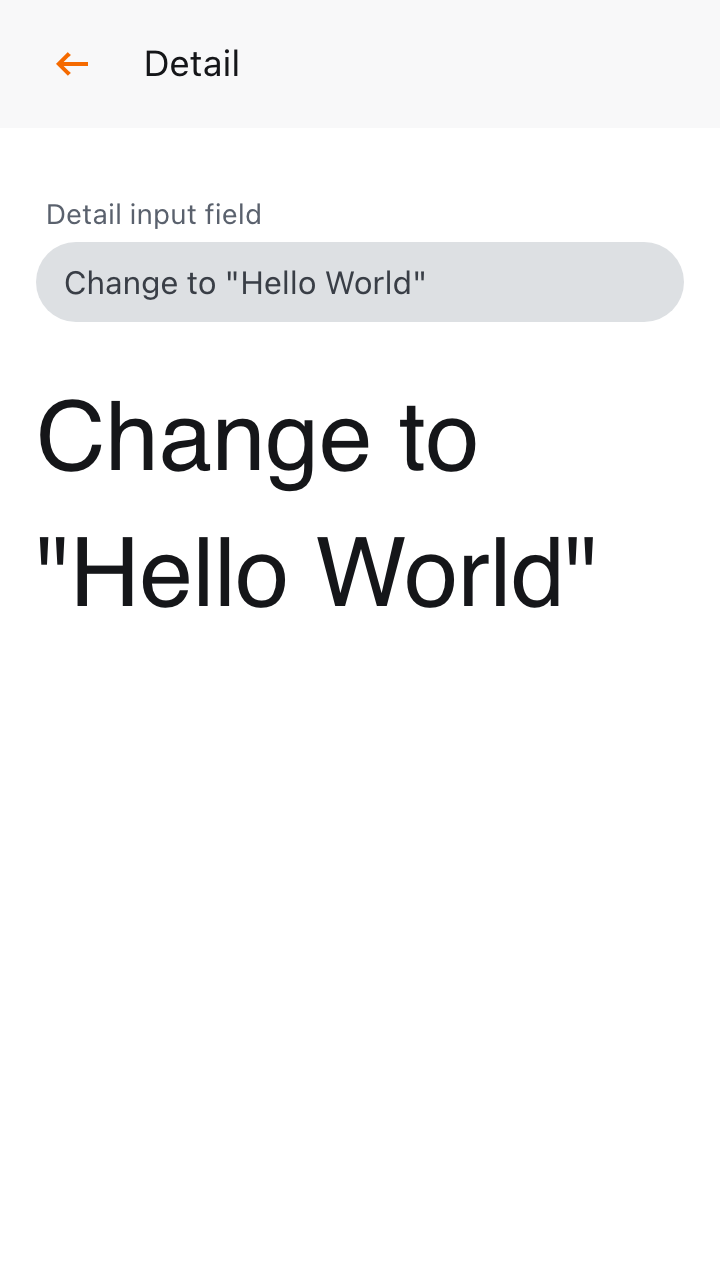
The result of Detail page in mobile browser with light theme
Using mobile browser with dark theme
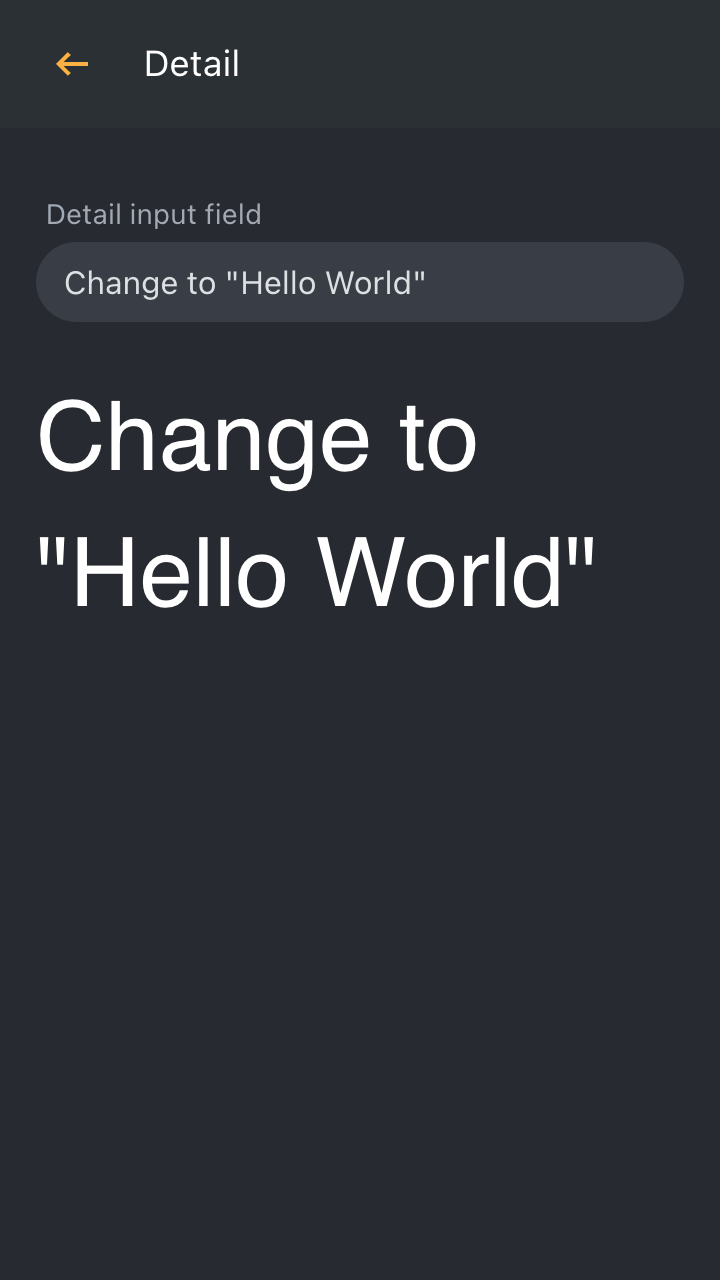
The result of Detail page in mobile browser with dark theme
Using mobile with light theme
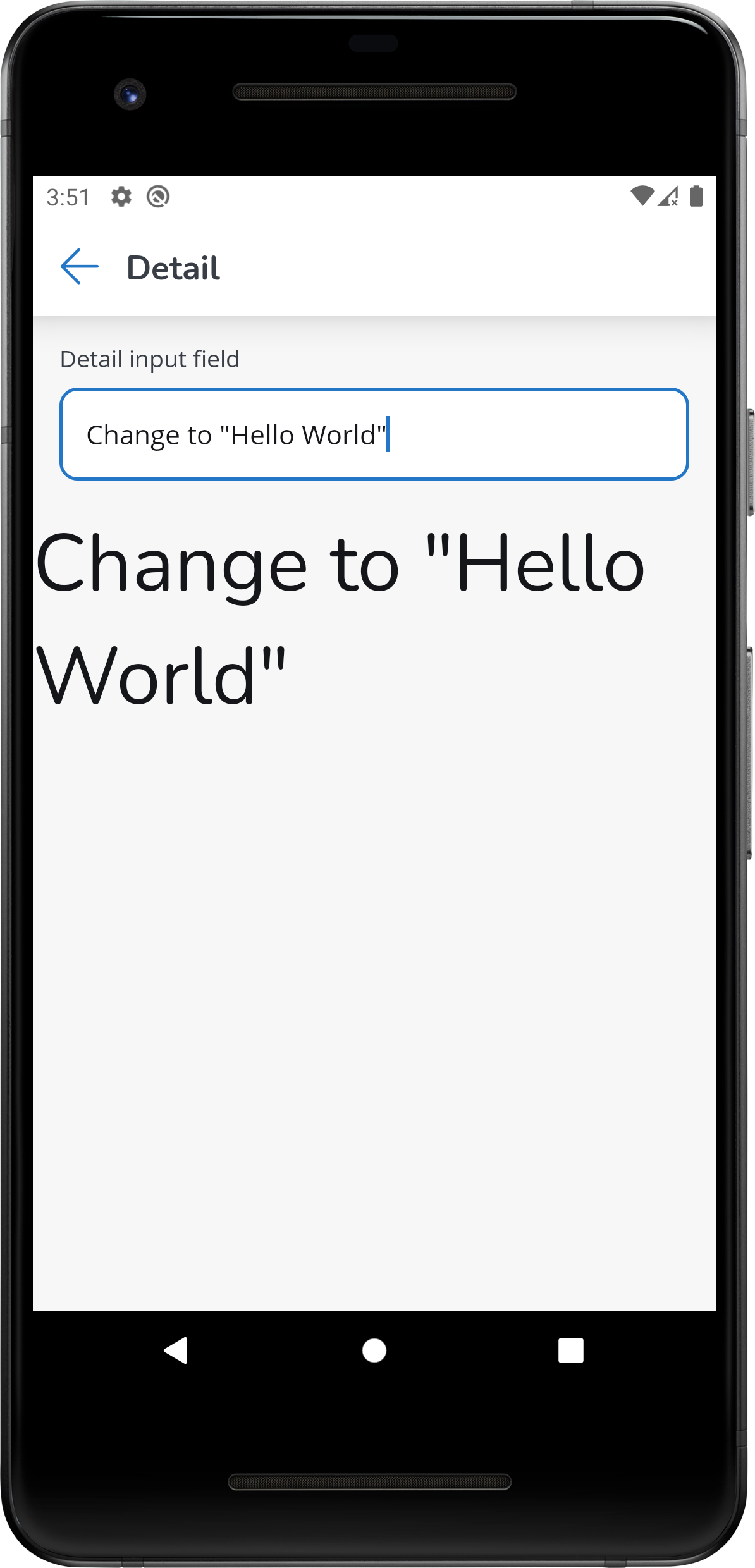
The result of Detail page in mobile with light theme
Using mobile with dark theme
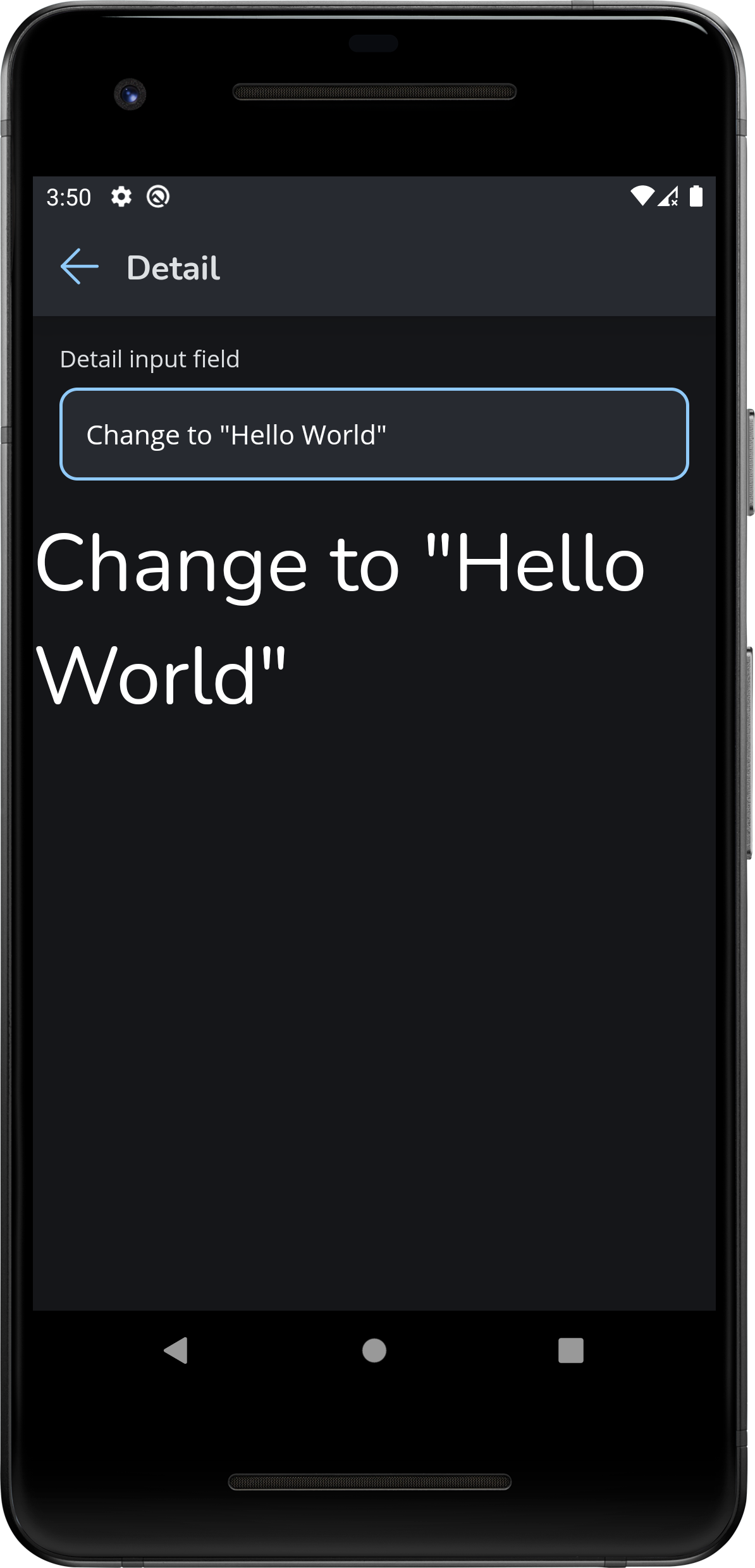
The result of Detail page in mobile with dark theme