List page with card template¶
In this lesson we will continue with our existing application use the code at the end of the previous lesson, where the goal is to display structured data in form of list using card format. (Details of the List element and list columns are described in the API documentation.)
Defining a list page¶
We extend the Notes page (created in the previous lesson) with a list populated with static data and displayed as a card.
Scripting hints¶
Small files: We split Scolvo scripts into separate files in order to keep it understandable for humans. In one file we usually keep same subdomain script parts together.
Naming convention: As finally all script segments will be built in one file it is of crucial importance to have good naming convention.
Global objects: Global variables and function names refer to page object e.g.: displayNotesPage.
Child element names in the page object: Page element names have strong relation to page’s name e.g.: NotesPageSaveButton.
Define separate display function with logic¶
As a first step we have created a more complex display function displayNotesPage to prepare data for the list page. We import the note specific functionality from the Notes.scolvo script file and use the displayNotesPage in the appropriate menu event.
Note
It is important to remove the old version of the NotesPage and corresponding on loaded function with name onNotesPageLoaded from the mobile.scolvo script to not interfere with the new definitions.
1import {
2 /mobile/note/Notes
3}
4
5// ...
6
7function onAll(originId) {
8 displayNotesPage(originId);
9}
10
11// ...
In the Notes.scolvo script we have prepared static data for the pageDescriptor object. The structure of this object follows the definition of the page object. NotesPage contains a list named NotesList where the template is listVerticalNormal and the itemTemplate is listItemCard. The listItemCard type uses two attributes: title and text. We have indicated the mapping of these attributes to the keys of the data structure (title, content).
1function displayNotesPage(originId) {
2 var pageData = getData();
3 var pageDescriptor = {
4 "NotesPage": {
5 "NotesList": pageData
6 }
7 };
8 display(NotesPage, pageDescriptor, originId);
9}
10
11function getData() {
12 return [{"title": "First title","content": "First content"},
13 {"title": "Second title","content": "Second content"}];
14}
15
16page NotesPage {
17 layout: vertical;
18 template: general;
19 settingsVisible: true;
20 scolvoMenuVisible: true;
21
22 list NotesList {
23 template: listVerticalNormal;
24 itemTemplate: listItemCard;
25 span: 0;
26 filterVisible: true;
27
28 actions: []
29 columns: [
30 title => title,
31 text => content
32 ]
33 }
34}
35
36function onNotesPageLoaded(originId) {}
Expected behavior after compile¶
After completing this code, while pressing the menu item All we will have a list displaying two note item cards.
Using browser with light theme
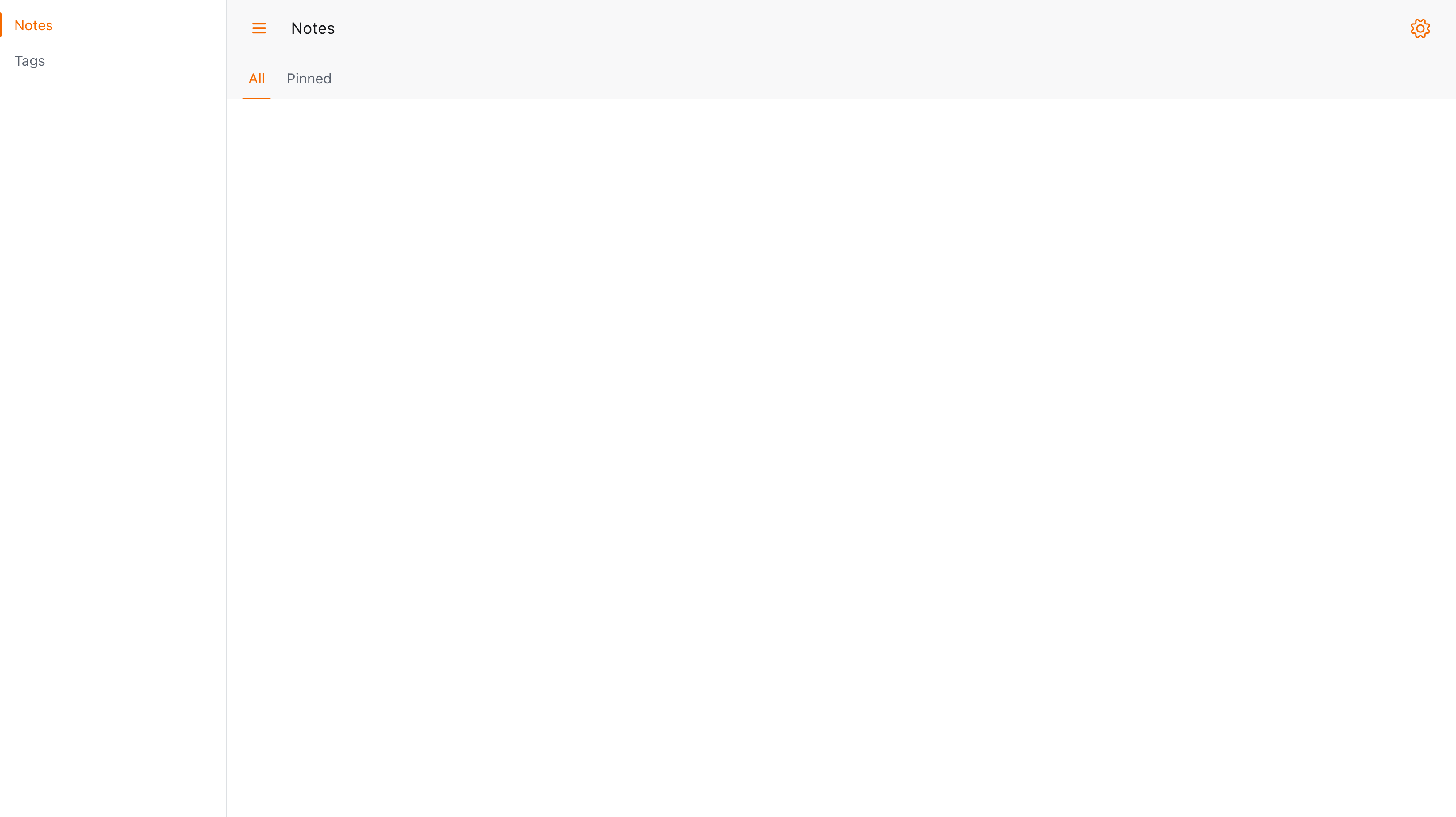
The result of code in browser with light theme
The final code with list page card template¶
After performing the changes described above, the final form of the mobile.scolvo and Notes.scolvo file should be as defined below:
1import {
2 /mobile/note/Notes
3}
4
5menu defaultItem Notes {
6 group Notes defaultItem All {
7 item All,
8 item Pinned
9 },
10 item Tags
11}
12
13function onAll(originId) {
14 displayNotesPage(originId);
15}
16
17function onPinned(originId) {
18 display(PinnedPage, {}, originId);
19}
20
21function onTags(originId) {
22 display(TagsPage, {}, originId);
23}
24
25page PinnedPage {
26 layout: vertical;
27 template: general;
28 settingsVisible: true;
29 scolvoMenuVisible: true;
30}
31
32function onPinnedPageLoaded(originId) {}
33
34page TagsPage {
35 layout: vertical;
36 template: general;
37 settingsVisible: true;
38 scolvoMenuVisible: true;
39}
40
41function onTagsPageLoaded(originId) {}
1function displayNotesPage(originId) {
2 var pageData = getData();
3 var pageDescriptor = {
4 "NotesPage": {
5 "NotesList": pageData
6 }
7 };
8 display(NotesPage, pageDescriptor, originId);
9}
10
11function getData() {
12 return [{"title": "First title","content": "First content"},
13 {"title": "Second title","content": "Second content"}];
14}
15
16page NotesPage {
17 layout: vertical;
18 template: general;
19 settingsVisible: true;
20 scolvoMenuVisible: true;
21
22 list NotesList {
23 template: listVerticalNormal;
24 itemTemplate: listItemCard;
25 span: 0;
26 filterVisible: true;
27
28 actions: []
29 columns: [
30 title => title,
31 text => content
32 ]
33 }
34}
35
36function onNotesPageLoaded(originId) {}
The content of the dictionary file is as below:
1menu_Notes_label=Notes
2menu_Notes_icon=\ue956
3menu_Notes_All_label=All
4menu_Notes_Pinned_label=Pinned
5menu_Tags_label=Tags
6menu_Tags_icon=\ue93b