Menu Structure lesson¶
In this lesson we start with a new application, so no previous code content is required. Deatils of menu item is described in Menu API documentation section.
Defining a menu with one item¶
Every application must have a menu defined, what is presented as entry point of the UI. At first we define a simple menu with only one Item, what is referenced as default item of the application.
1menu defaultItem Tags {
2 item Tags
3}
For the new menu item we define a label and an icon (check the Available icons page) too:
1menu_Tags_label=Tags
2menu_Tags_icon=\ue93b
When a user selects a menu item, then a function with name on<Item name> is executed. For our example this function is the onTags(originId).
1function onTags(originId) {
2}
We can define a Page object to be presented when selecting this Tags menu.
1page TagsPage {
2 layout: vertical;
3 template: general;
4 settingsVisible: true;
5 scolvoMenuVisible: true;
6}
7
8function onTagsPageLoaded(originId) {}
Now we can change the logic of the onTags function to open the Tags page using the display() built-in function.
1function onTags(originId) {
2 display(TagsPage, {}, originId);
3}
Extending the menu with a group¶
To have more sophisticated application we introduce a menu group. So the new form of the menu definition is as below:
1menu defaultItem Notes {
2 group Notes defaultItem All {
3 item All
4 },
5 item Tags
6}
We extend the dictionary with new key (for an item in group only the label can be defined):
1menu_Notes_All_label=All
The default item of the menu is now the Notes group, and as the default item of the Notes is the All item, this is the default of the menu.
For the new All item a function is defined too:
1function onAll(originId) {
2}
To have meaningful example we add a page to be opened and change the content of the onAll() function.
1page NotesPage {
2 layout: vertical;
3 template: general;
4 settingsVisible: true;
5 scolvoMenuVisible: true;
6}
7
8function onNotesPageLoaded(originId) {}
9
10function onAll(originId) {
11 display(NotesPage, {}, originId);
12}
To have more complex functionality we extend the group with another item, Pinned.
1menu defaultItem Notes {
2 group Notes defaultItem All {
3 item All,
4 item Pinned
5 },
6 item Tags
7}
Now we can define the label for the Pinned page:
1menu_Notes_Pinned_label=Pinned
Another page is defined for this menu item with name PinnedPage. The code now contains the onPinned() function and the page definition too:
1page PinnedPage {
2 layout: vertical;
3 template: general;
4 settingsVisible: true;
5 scolvoMenuVisible: true;
6}
7
8function onPinnedPageLoaded(originId) {}
9
10function onPinned(originId) {
11 display(PinnedPage, {}, originId);
12}
The final code with menu items and groups¶
After performing the changes descibed above, the final form of the mobile.scolvo file should be as defined below:
1menu defaultItem Notes {
2 group Notes defaultItem All {
3 item All,
4 item Pinned
5 },
6 item Tags
7}
8
9function onAll(originId) {
10 display(NotesPage, {}, originId);
11}
12
13function onPinned(originId) {
14 display(PinnedPage, {}, originId);
15}
16
17function onTags(originId) {
18 display(TagsPage, {}, originId);
19}
20
21page NotesPage {
22 layout: vertical;
23 template: general;
24 settingsVisible: true;
25 scolvoMenuVisible: true;
26}
27
28function onNotesPageLoaded(originId) {}
29
30page PinnedPage {
31 layout: vertical;
32 template: general;
33 settingsVisible: true;
34 scolvoMenuVisible: true;
35}
36
37function onPinnedPageLoaded(originId) {}
38
39page TagsPage {
40 layout: vertical;
41 template: general;
42 settingsVisible: true;
43 scolvoMenuVisible: true;
44}
45
46function onTagsPageLoaded(originId) {}
The content of the dictionary file is as below:
1menu_Notes_label=Notes
2menu_Notes_icon=\ue956
3menu_Notes_All_label=All
4menu_Notes_Pinned_label=Pinned
5menu_Tags_label=Tags
6menu_Tags_icon=\ue93b
Menu structure screen shots¶
The UI resulted of the above code on different client types should look as below:
Using browser with light theme
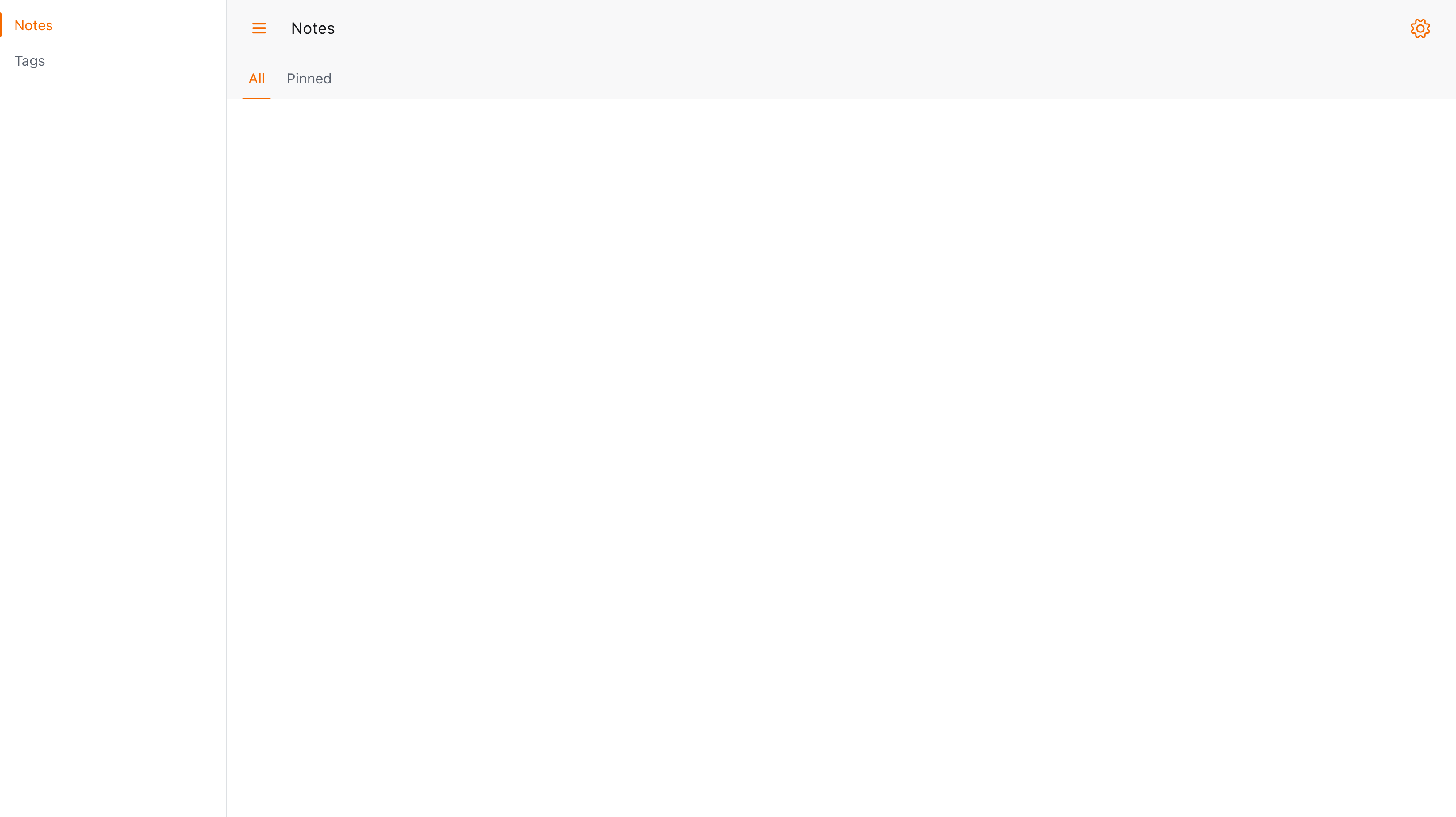
The result of code in browser with light theme
Using browser with dark theme
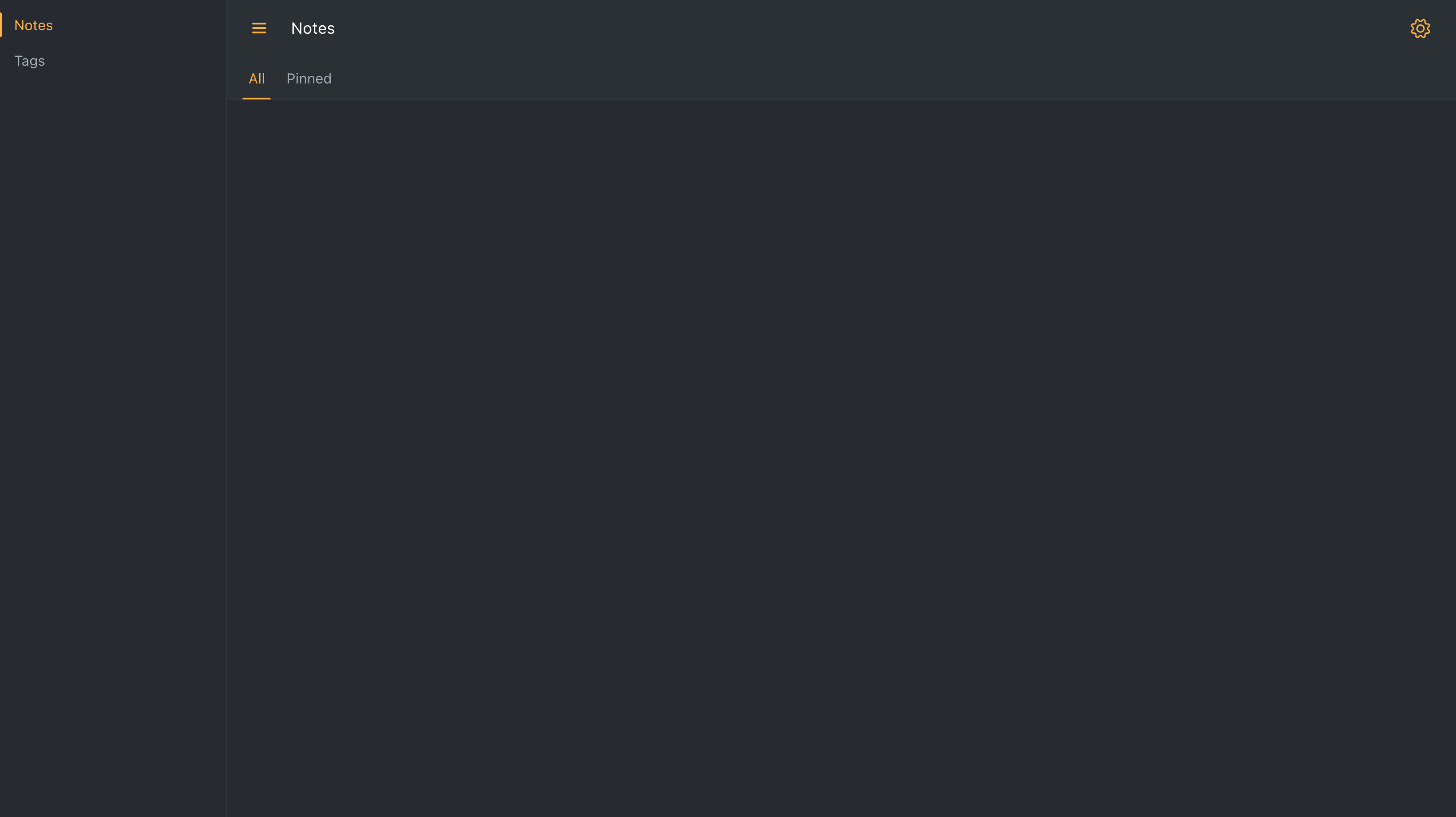
The result of code in browser with dark theme
Using mobile browser with light theme
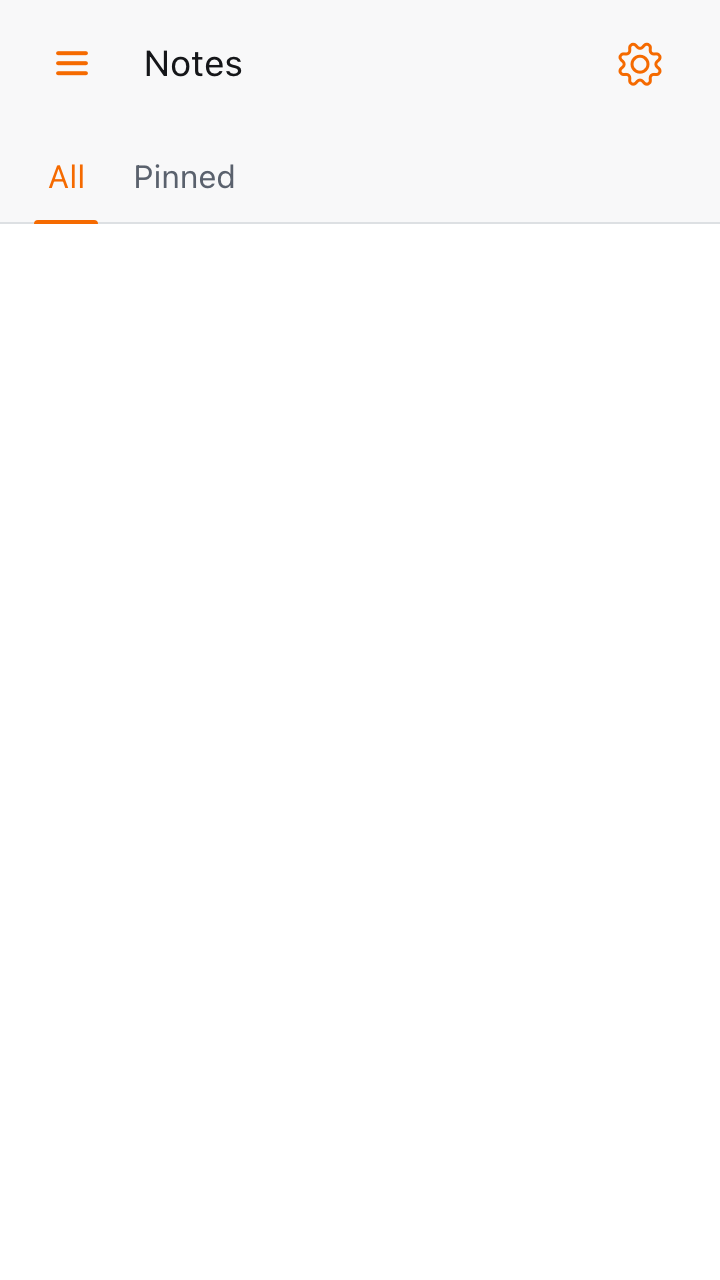
The result of code in mobile browser with light theme
Using mobile browser with dark theme
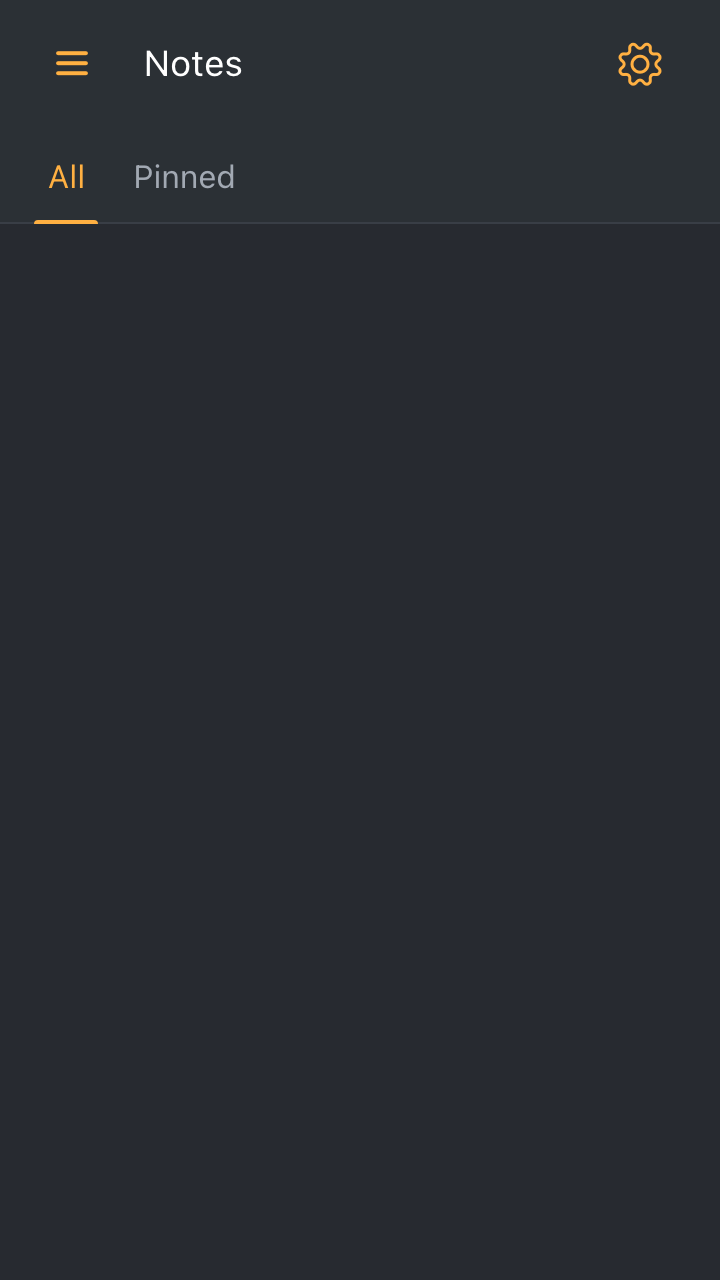
The result of code in mobile browser with dark theme
Using mobile with light theme
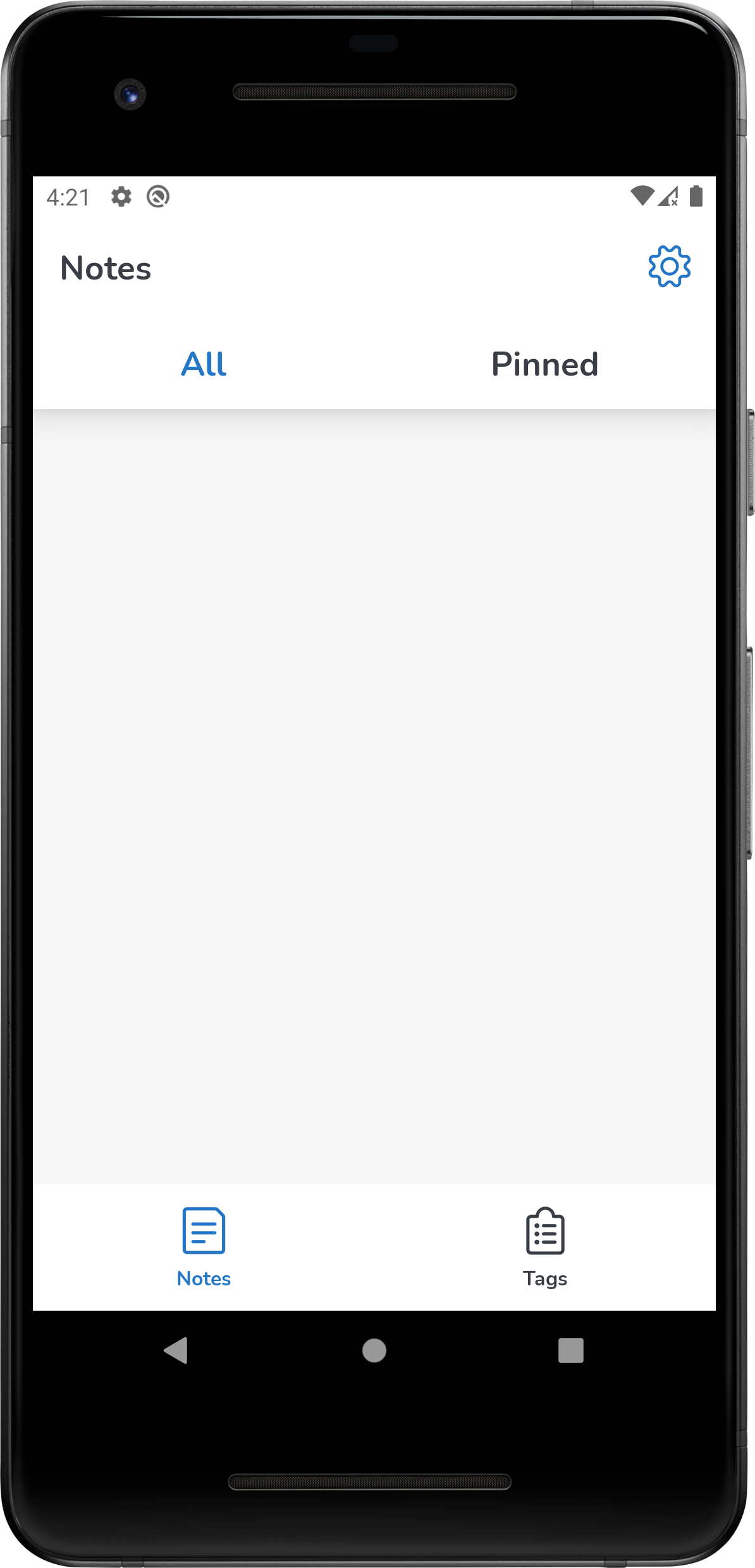
The result of code mobile with light theme
Using mobile with dark theme
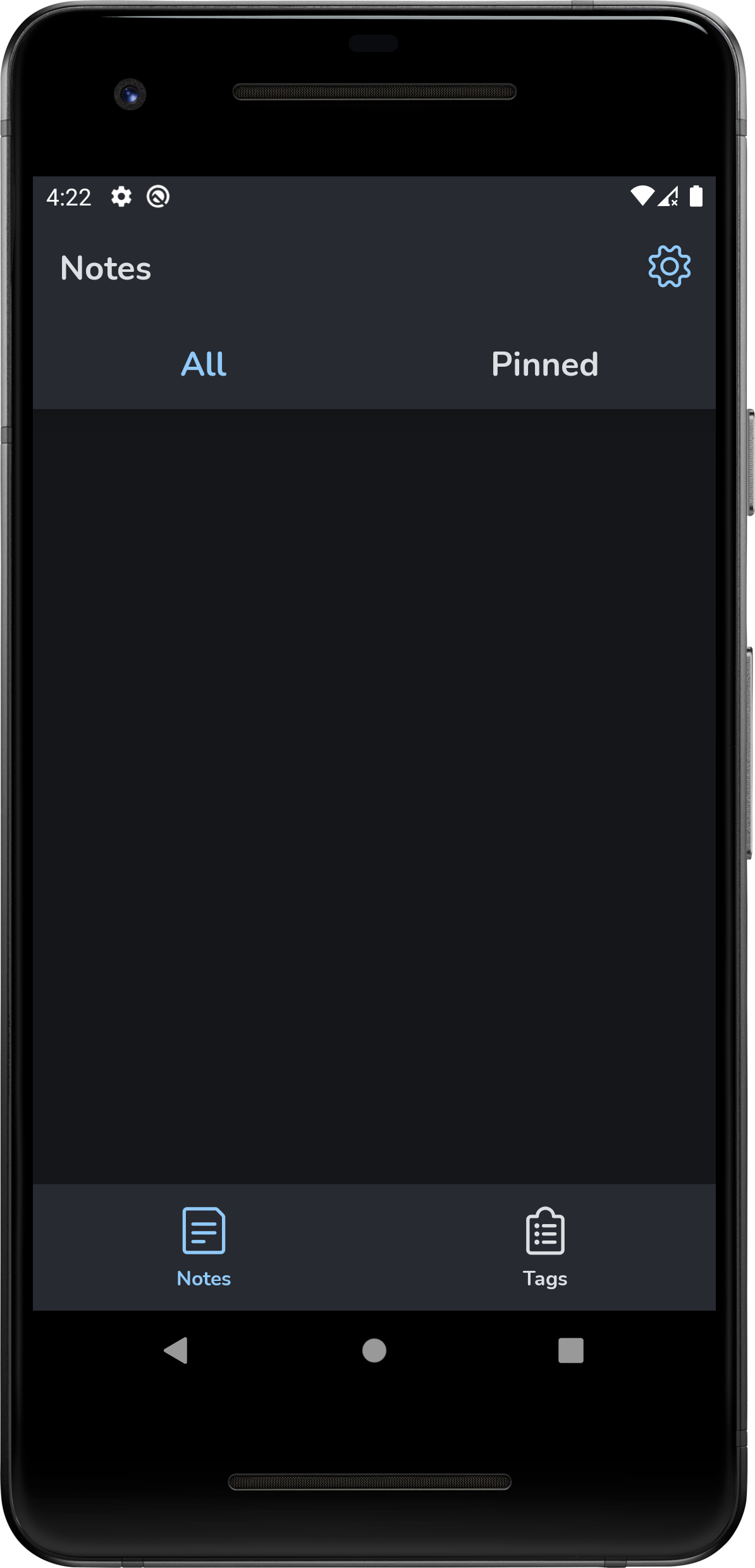
The result of code in mobile with dark theme